Getting started
Mantine ContextMenu depends on @mantine/core
, @mantine/hooks
and clsx
.
Create a new application with Mantine, install mantine-contextmenu
and make sure you have the clsx
dependency installed as well:
Wrap your application in a ContextMenuProvider
inside the MantineProvider
and don’t forget to import the necessary CSS files in the correct order.
For example, if you’re using a Next.js application with an app router, your layout.tsx
could look like this:
Use the hook in your code
Import the useContextMenu
hook and use it in your components like so:
The above code will produce the following result (right-click on the image, or long-tap on mobile devices, to trigger the context menu):
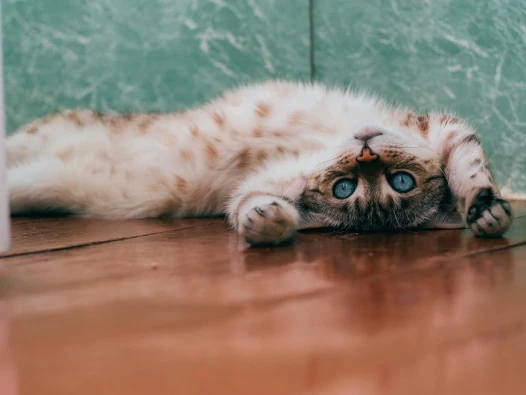
Picture by Daria Shatova
Next, let’s make sure you have a good understanding of how styling works before browsing the usage examples and taking a look at the type definitions page.