Examples › Submenus
An item with an items
property will render a submenu, available when hovering over the given option.
Right-click on the image to trigger the context menu:
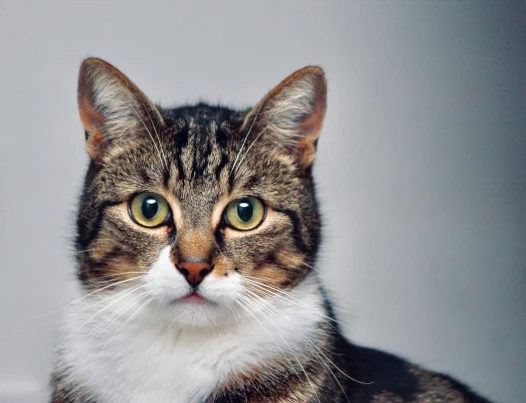
Picture by Lloyd Henneman
Nesting
You can nest as many submenus as you want. Don’t abuse this feature, though, as it might be confusing for the user.
Right-click on the image to trigger the context menu:
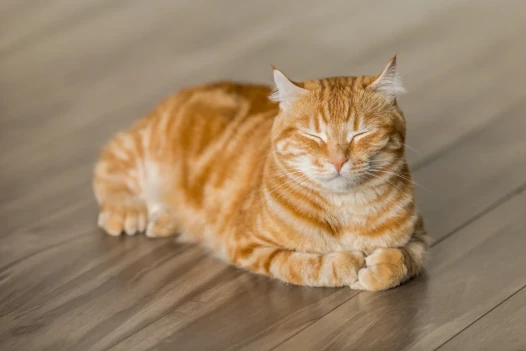
Picture by Michael Sum
Controlling the show/hide delay
You can change the default 500ms
delay between hovering over or out of an item and the submenu appearing or disappearing by setting the submenuDelay
property of the ContextMenuProvider
component.
Right-click on the image to trigger the context menu:
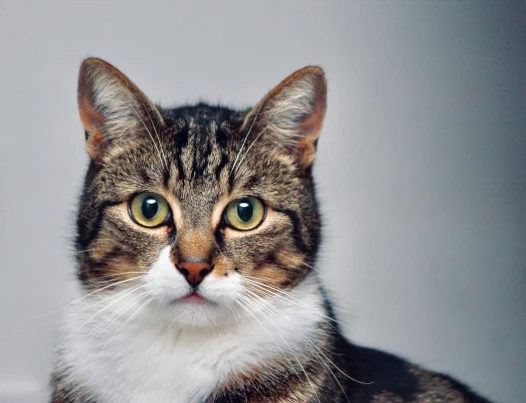
Picture by Lloyd Henneman
Using a custom arrow icon
You can customize the submenu arrow icon by setting the iconRight
property like in the following example:
Right-click on the image to trigger the context menu:
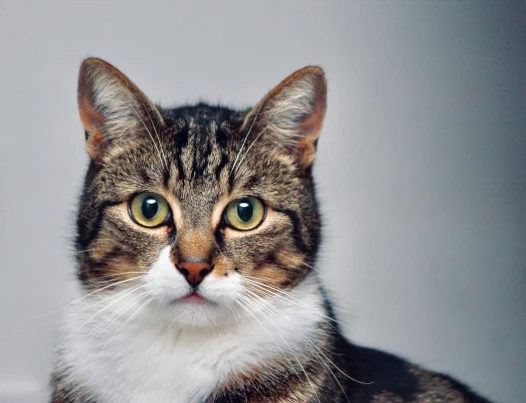
Picture by Lloyd Henneman
Head over to the next example to discover other features.