Examples › Imperative hiding
A visible context menu hides automatically when the user clicks anywhere on the page, hits the Escape
key, scrolls the or resizes the browser window.
However, you can also hide the context menu imperatively by calling the hideContextMenu
function returned by the useContextMenu
hook.
The useContextMenu
hook also returns an isContextMenuVisible
boolean that you can use to determine whether the context menu is currently visible.
In the example below, we’ll hide the context menu automatically when the user presses the H
key, his mouse cursor leaves the page, or after five seconds have elapsed:
Right-click on the image below to show the context menu:
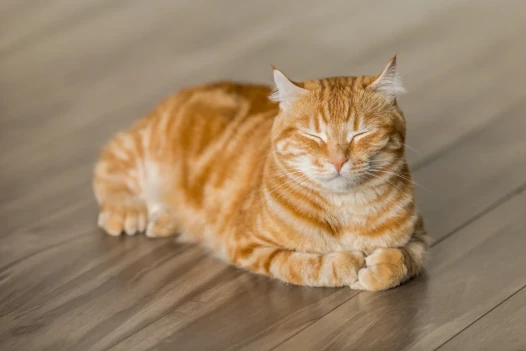
Picture by Michael Sum