Examples › Custom styling
There are more ways to style a context menu besides setting its basic configuration options and its items action colors.
The ContextMenuProvider
wrapper component accepts the following properties: className
, style
, classNames
and styles
.
Have a look at Mantine Styles API documentation to make a general idea of how these properties work.
The className
and style
properties can be used to target the component root, while the classNames
and styles
properties can be used to target the individual component parts, root
, item
and divider
.
If you provide the above properties to the ContextMenuProvider
component, they will be passed down to all the context menus that are rendered within the provider.
The ContextMenuProvider
styling values can be overriden by setting the similarly-named properties of an options object passed as the second argument to the showContextMenu
function returned by useContextMenu
hook to target the individual context menu instances, in which case they will override the initial provider properties.
The examples below will use this approach.
Styling the container with a className
You can specify a className
that will target the component root:
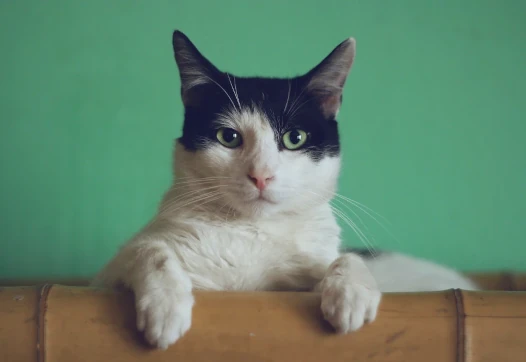
Picture by Manja Vitolic
Styling the container with a style object
You can specify a style
object with CSS properties that will be applied to the component root:
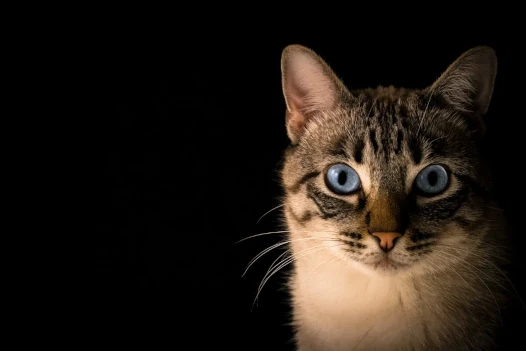
Picture by Pacto Visual
The style
property can also point to a function that will receive the current theme as an argument and return a style object:
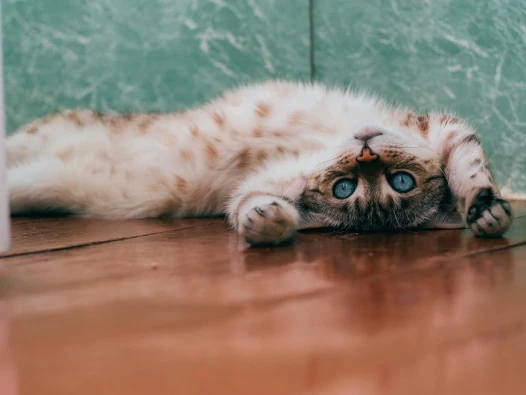
Picture by Daria Shatova
Styling with multiple class names
Here’s an example of how you can use classNames
property to target the individual component parts:
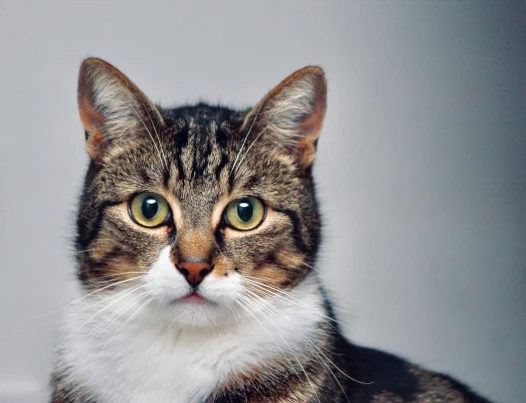
Picture by Lloyd Henneman
Styling with multiple style objects
Here’s an example of how you can use the styles
property to target the individual component parts:
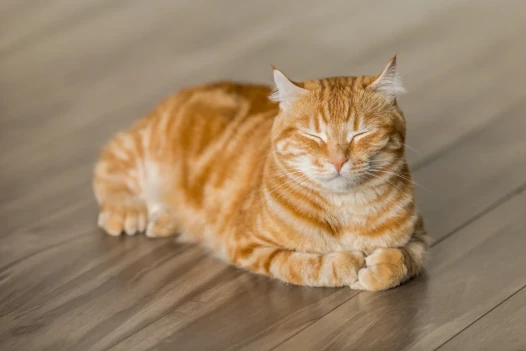
Picture by Michael Sum
You can also use functions that receive the current theme as an argument and return styles objects:
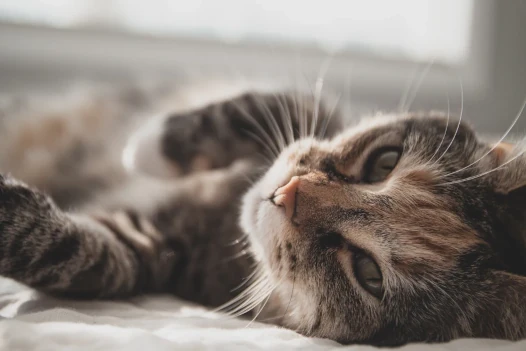
Picture by Zeke Tucker
Styling individual actions with className and style
You can also style individual actions by passing a className
or style
property to the object describing an individual menu item:
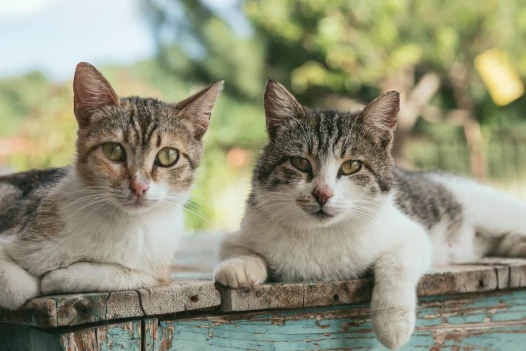
Picture by Nathalie Jolie
Head over to the next example to discover other features.